What is Git? Why Git?
Why Git for your organization
Feature Branch Workflow: One of the biggest advantages of Git is its branching capabilities. Unlike centralized version control systems, Git branches are cheap and easy to merge. This facilitates the feature branch workflow popular with many Git users.
Distributed Development: In SVN, each developer gets a working copy that points back to a single central repository. Git, however, is a distributed version control system. Instead of a working copy, each developer gets their own local repository, complete with a full history of commits.
Pull Requests: A pull request is a way to ask another developer to merge one of your branches into their repository. This not only makes it easier for project leads to keep track of changes, but also lets developers initiate discussions around their work before integrating it with the rest of the codebase.
Faster Release Cycle: The ultimate result of feature branches, distributed development, pull requests, and a stable community is a faster release cycle. These capabilities facilitate an agile workflow where developers are encouraged to share smaller changes more frequently. In turn, changes can get pushed down the deployment pipeline faster than the monolithic releases common with centralized version control systems.
什么是版本控制?
为什么要使用版本控制系统?
命令行界面还是图形界面?
为什么选择 Git
Installation
Install Git Directly
Getting Started - Installing Git
Install Git - Atlassian(https://www.atlassian.com/git/tutorials/install-git)
Install Git through Git Client
Git GUI Clients Guide:GUI Clients
Tutorial
Getting Git Right - Atlassian
Learn Version Control with Git - Tower
git - the simple guide
Git Cheat Sheet
https://www.git-tower.com/learn/cheat-sheets/git
进阶
Inspecting a repository
git tag
Tags are ref’s that point to specific points in Git history. git tag
is generally used to capture a point in history that is used for a marked version release (i.e. v1.0.1).
具体使用
Lightweight tags and Annotated tags:
Annotated tags store extra meta data such as: the tagger name, email, and date. This is important data for a public release. Lightweight tags are essentially ‘bookmarks’ to a commit, they are just a name and a pointer to a commit, useful for creating quick links to relevant commits.
创建 tag
1 | git tag <tagname> |
创建 Annotated Tags
1 | git tag -a v1.4 |
查看所有 tag
1 | git tag |
查看筛选后的 tag
1 | git tag -l *-rc* |
默认情况下打的 tag 是在当前 HEAD 所在的 commit 上,若要在其他的 commit 上打 tag,需要加上 commit SHA hash
1 | git tag -a v1.2 15027957951b64cf874c3557a0f3547bd83b3ff6 |
替换已有的 tag:默认情况下打重复的 tag 是会报错的,这时候需要加上
-f FORCE
1 | git tag -a -f v1.4 15027957951b64cf874c3557a0f3547bd83b3ff6 |
将 tag 推送到远程仓库
1 | git push origin v1.4 |
删除 tag
1 | $ git tag |
git blame
The high-level function of git blame
is the display of author metadata attached to specific committed lines in a file. This is used to explore the history of specific code and answer questions about what, how, and why the code was added to a repository.
git log
The git log
command displays committed snapshots. It lets you list the project history, filter it, and search for specific changes.
git log
与 git status
的区别
具体使用
通过
<limit>
限制展示的个数。1 | git log -n <limit> |
将每个 commit 的信息压缩为一行展示。
1 | git log --oneline |
展示每一次 commit 中哪些文件变更及具体的行数。
1 | git log --stat |
展示每一次 commit 完整的变更内容。
1 | git log -p |
展示某个开发者的 commit,
<pattern>
可以是字符串或者正则。1 | git log --author="<pattern>" |
👍 搜索 commit message 匹配
<pattern>
的 commits 并展示,<pattern>
可以是字符串或者正则。1 | git log --grep="<pattern>" |
只展示在
<since>
和 <until>
之间的提交,<since>
和 <until>
可以是:commit ID, a branch name, HEAD, or any other kind of revision reference.1 | git log <since>..<until> |
👍 只查看某个文件的提交历史。
1 | git log -- <file> |
👍 通过内容搜索。
1 | git log -G"<regex>" |
A few useful options to consider. The –graph flag that will draw a text based graph of the commits on the left hand side of the commit messages. –decorate adds the names of branches or tags of the commits that are shown. –oneline shows the commit information on a single line making it easier to browse through commits at-a-glance.
1 | git log --graph --decorate --oneline |
Merging vs. Rebasing
Both of these commands are designed to integrate changes from one branch into another branch—they just do it in very different ways.
git rebase
的优缺点
The major benefit of rebasing is that you get a much cleaner project history.
There are two trade-offs for this pristine commit history: safety and traceability.
The Merge Option
The Merge Option
1 | git checkout feature |
git merge
的好处在于它是一个非破坏性(non-destructive)的操作,保留了变更历史,但同时也增加了多余的 commit。
The Rebase Option
The Rebase Option
1 | git checkout feature |
Interactive Rebasing
Interactive Rebasing
Interactive Rebasing 使你能够在 rebase 之前将你的 commit 历史做修改。
1 | # To begin an interactive rebasing session, pass the i option to the git rebase command: |
Project history that looks like the following:
The Golden Rule of Rebasing
⚠️ Never use it on public branches.
How to Squash Commits in Git
How to Squash Commits in Git | Learn Version Control with Git - https://www.git-tower.com/learn/git/faq/git-squash/
1 | git rebase -i HEAD~3 |
Resetting, Checking Out & Reverting
git reset
、git checkout
、git revert
all let you undo some kind of change in your repository, and the first two commands can be used to manipulate either commits or individual files.
- A checkout is an operation that moves the HEAD ref pointer to a specified commit.
- A revert is an operation that takes a specified commit and creates a new commit which inverses the specified commit.
- A reset is an operation that takes a specified commit and resets the “three trees” to match the state of the repository at that specified commit.
Checkout and reset are generally used for making local or private 「undos」. They modify the history of a repository that can cause conflicts when pushing to remote shared repositories. Revert is considered a safe operation for 「public undos」 as it creates new history which can be shared remotely and doesn’t overwrite history remote team members may be dependent on.
“The three trees” of Git.
Git Reset vs Revert vs Checkout reference
Command | Scope | Common use cases |
---|---|---|
git reset |
Commit-level | Discard commits in a private branch or throw away uncommited changes |
git reset |
File-level | Unstage a file |
git checkout |
Commit-level | Switch between branches or inspect old snapshots |
git checkout |
File-level | Discard changes in the working directory |
git revert |
Commit-level | Undo commits in a public branch |
git revert |
File-level | (N/A) |
Example
Reset A Specific Commit
The following command moves the
hotfix
branch backwards by two commits.1 | git checkout hotfix |
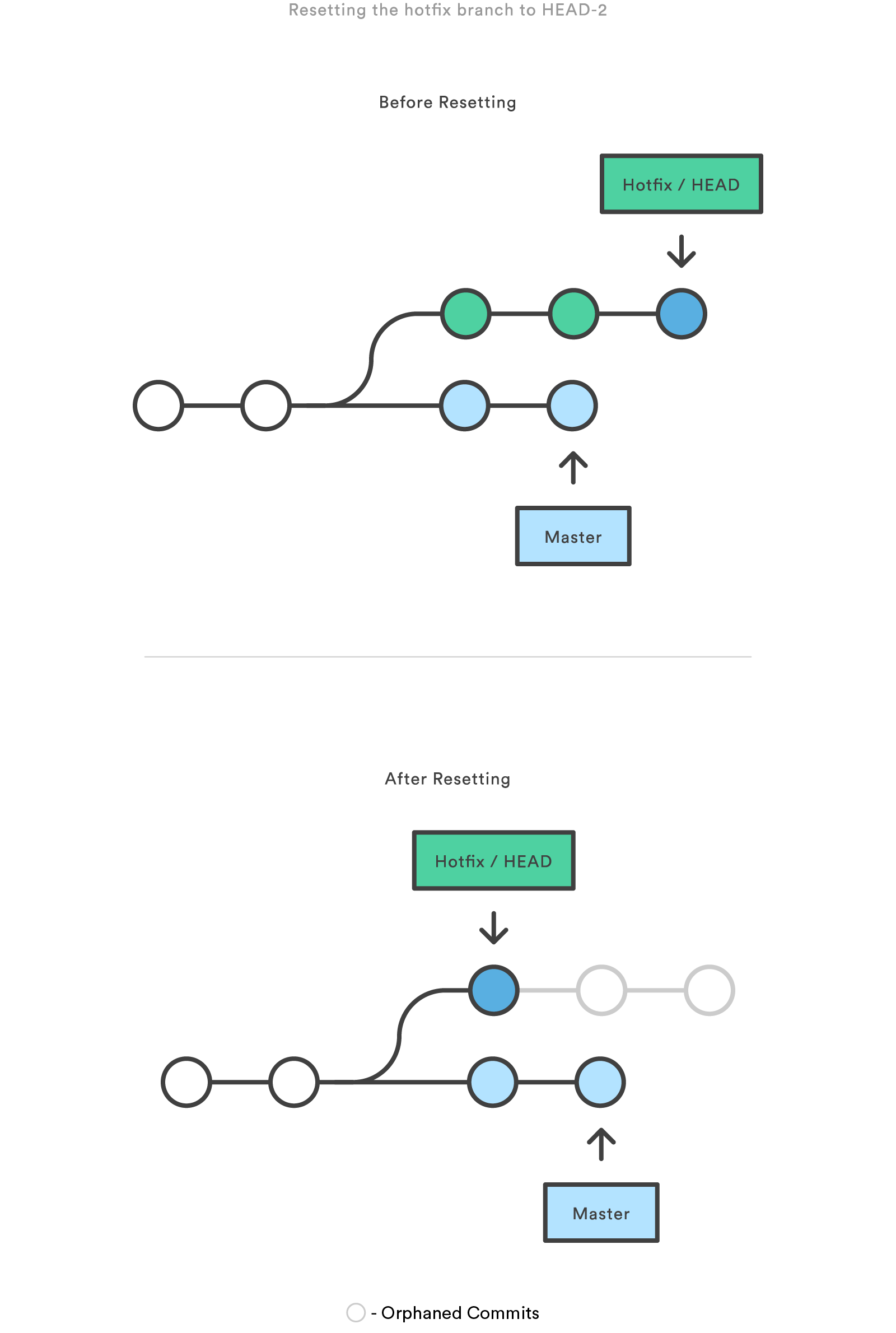
Checkout old commits
When passed with a branch name, it lets you switch between branches.
1 | git checkout hotfix8 |
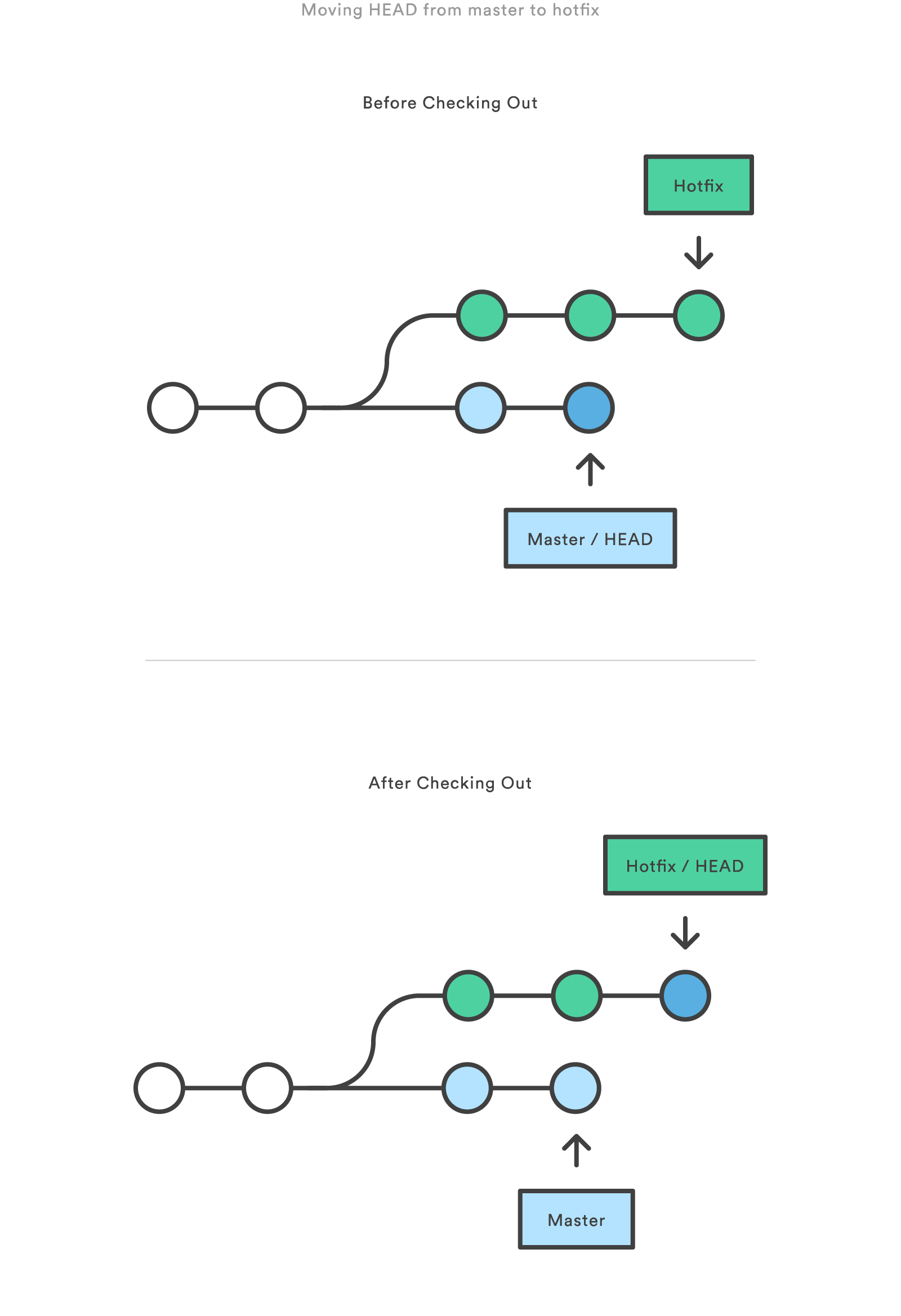
You can also check out arbitrary commits by passing the commit reference instead of a branch.
1 | git checkout HEAD~2 |
Undo Public Commits with Revert
The following command will figure out the changes contained in the 2nd to last commit, create a new commit undoing those changes, and tack the new commit onto the existing project.
1 | git checkout hotfix |
Summary
You can also think of git revert as a tool for undoing committed changes, while git reset HEAD is for undoing uncommitted changes.
Git Hooks
Git hooks are scripts that run automatically every time a particular event occurs in a Git repository. They let you customize Git’s internal behavior and trigger customizable actions at key points in the development life cycle.
Hooks can reside in either local or server-side repositories, and they are only executed in response to actions in that repository.
Local Hooks
6 of the most useful local hooks:
- pre-commit
- prepare-commit-msg
- commit-msg
- post-commit
- post-checkout
- pre-rebase
The first 4 hooks let you plug into the entire commit life cycle, and the final 2 let you perform some extra actions or safety checks for the git checkout and git rebase commands, respectively.
All of the pre- hooks let you alter the action that’s about to take place, while the post- hooks are used only for notifications.
Server-side Hooks
- pre-receive
- update
- post-receive
All of these hooks let you react to different stages of the git push
process.
Pre-Receive
The pre-receive hook is executed every time somebody uses git push to push commits to the repository. It should always reside in the remote repository that is the destination of the push, not in the originating repository.
Update
The update
hook is called after pre-receive
, and it works much the same way. It’s still called before anything is actually updated, but it’s called separately for each ref that was pushed. That means if the user tries to push 4 branches, update
is executed 4 times. Unlike pre-receive
, this hook doesn’t need to read from standard input. Instead, it accepts the following 3 arguments:
- The name of the ref being updated
- The old object name stored in the ref
- The new object name stored in the ref
Post-Receive
The post-receive
hook gets called after a successful push operation, making it a good place to perform notifications. For many workflows, this is a better place to trigger notifications than post-commit
because the changes are available on a public server instead of residing only on the user’s local machine. Emailing other developers and triggering a continuous integration system are common use cases for post-receive.
Git Cherry Pick
git cherry-pick
is a powerful command that enables arbitrary Git commits to be picked by reference and appended to the current working HEAD. Cherry picking is the act of picking a commit from a branch and applying it to another. git cherry-pick
can be useful for undoing changes. For example, say a commit is accidently made to the wrong branch. You can switch to the correct branch and cherry-pick the commit to where it should belong.
迁移 Git 仓库
How to move a git repository with history | Atlassian Git Tutorial
Tips & Tricks
git hook
使用 Tower 提高 Git 效率
使用 Tower 提高 Git 效率
Tower Help for Mac 『 Tower 文章导航 』
Keyboard Shortcuts 『 Tower 常用快捷键 』
Tips & Tricks 『 Tower 使用技巧 』
A Basic Workflow 『 Tower 使用流程 』
Git Flow
A successful Git branching model
SSH
SSH (SECURE SHELL) 『 SSH 官网的介绍 』
Relevant Infomations
Relevant Terminology,Git Commands Glossary
git-cheat-sheet
Command Line Cheat Sheet
Version Control Best Practices,中文版
Authentication with SSH Public Keys
Handling Large Files with LFS
Workflows with git-flow
Pro Git 『 Pro Git 在线书籍 』
git-cheat-sheet
从 Subversion 过渡到 Git